Python依赖注入框架Injector的下载与安装教程
2024-12-17 17:29:07作者:韦蓉瑛
1. 项目介绍
Python的依赖注入框架Injector,是一款受到Guice启发的开源项目。它旨在简化Python中的依赖注入过程,通过自动提供依赖关系,减少大型应用程序中的模板代码。Injector框架鼓励使用模块化的代码编写方式,它不仅减少了代码间的耦合,还支持静态类型检查。
2. 项目下载位置
本项目托管在GitHub上,项目地址为:https://github.com/python-injector/injector.git
。
3. 项目安装环境配置
在开始安装前,请确保您的系统中已安装Python。以下步骤以Windows系统为例,展示配置环境的过程:

图片示例中应包含Python版本信息,以及已安装的pip等必要工具。
4. 项目安装方式
项目可以通过以下两种方式进行安装:
使用pip安装
最简单的方式是使用pip命令直接安装:
pip install injector
从源代码安装
如果您希望从源代码安装,可以按照以下步骤进行:
-
克隆项目到本地:
git clone https://github.com/python-injector/injector.git
-
进入项目目录,安装项目:
cd injector pip install .
5. 项目处理脚本
项目中的injector_test.py
为单元测试脚本,可以用来测试安装的正确性和功能实现。
运行测试脚本:
python injector_test.py
确保所有测试通过,以此来验证安装的正确性。
以上就是Python依赖注入框架Injector的下载与安装教程,您可以开始享受它带来的便利了。
热门项目推荐
相关项目推荐
topiam-eiam
开源IDaas/IAM平台,用于管理企业内员工账号、权限、身份认证、应用访问,帮助整合部署在本地或云端的内部办公系统、业务系统及三方 SaaS 系统的所有身份,实现一个账号打通所有应用的服务。Java00excelize
https://github.com/xuri/excelize Excelize 是 Go 语言编写的一个用来操作 Office Excel 文档类库,基于 ECMA-376 OOXML 技术标准。可以使用它来读取、写入 XLSX 文件,相比较其他的开源类库,Excelize 支持操作带有数据透视表、切片器、图表与图片的 Excel 并支持向 Excel 中插入图片与创建简单图表,目前是 Go 开源项目中唯一支持复杂样式 XLSX 文件的类库,可应用于各类报表平台、云计算和边缘计算系统。Go02每日精选项目
🔥🔥 推荐每日行业内最新、增长最快的项目,快速了解行业最新热门项目动态~ 🔥🔥017Cangjie-Examples
本仓将收集和展示高质量的仓颉示例代码,欢迎大家投稿,让全世界看到您的妙趣设计,也让更多人通过您的编码理解和喜爱仓颉语言。Cangjie037毕方Talon工具
本工具是一个端到端的工具,用于项目的生成IR并自动进行缺陷检测。Python038advanced-java
Advanced-Java是一个Java进阶教程,适合用于学习Java高级特性和编程技巧。特点:内容深入、实例丰富、适合进阶学习。JavaScript0100taro
开放式跨端跨框架解决方案,支持使用 React/Vue/Nerv 等框架来开发微信/京东/百度/支付宝/字节跳动/ QQ 小程序/H5/React Native 等应用。 https://taro.zone/TypeScript010Yi-Coder
Yi Coder 编程模型,小而强大的编程助手HTML012Community
Cangjie-TPC(Third Party Components)仓颉编程语言三方库社区资源汇总05- Bbrew🍺 The missing package manager for macOS (or Linux)Ruby01
热门内容推荐
最新内容推荐
项目优选
收起
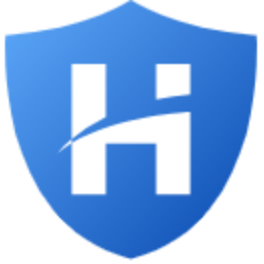
旨在打造算法先进、性能卓越、高效敏捷、安全可靠的密码套件,通过轻量级、可剪裁的软件技术架构满足各行业不同场景的多样化要求,让密码技术应用更简单,同时探索后量子等先进算法创新实践,构建密码前沿技术底座!
C
41
32
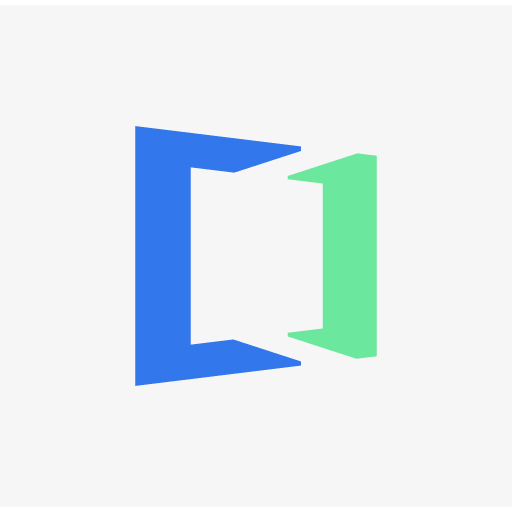
为仓颉编程语言开发者打造活跃、开放、高质量的社区环境
Markdown
891
0
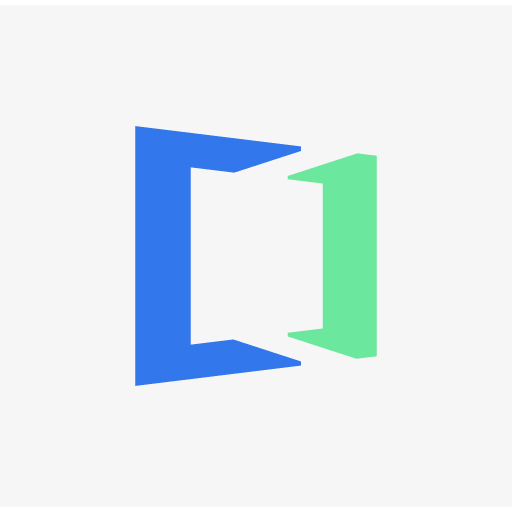
本仓将收集和展示高质量的仓颉示例代码,欢迎大家投稿,让全世界看到您的妙趣设计,也让更多人通过您的编码理解和喜爱仓颉语言。
Cangjie
163
37
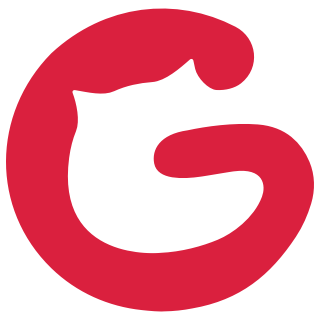
GitCode光引计划有奖征文大赛
16
1

🎉 基于SpringBoot,Spring Security,JWT,Vue & Element 的前后端分离权限管理系统,同时提供了 Vue3 的版本
Java
158
32
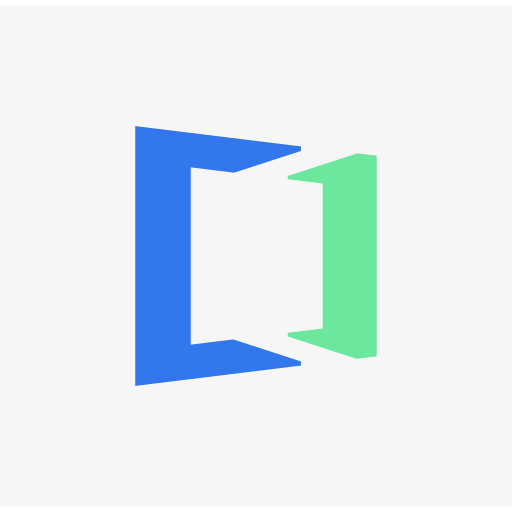
仓颉语言实现的Redis客户端SDK。已适配仓颉0.53.4 Beta版本。接口设计兼容jedis接口语义,支持RESP2和RESP3协议,支持发布订阅模式,支持哨兵模式和集群模式。
Cangjie
400
44

🎉 基于Spring Boot、Spring Cloud & Alibaba、Vue3 & Vite、Element Plus的分布式前后端分离微服务架构权限管理系统
Vue
20
15
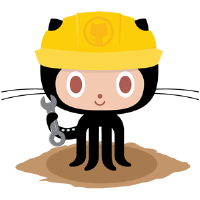
Advanced-Java是一个Java进阶教程,适合用于学习Java高级特性和编程技巧。特点:内容深入、实例丰富、适合进阶学习。
JavaScript
377
100
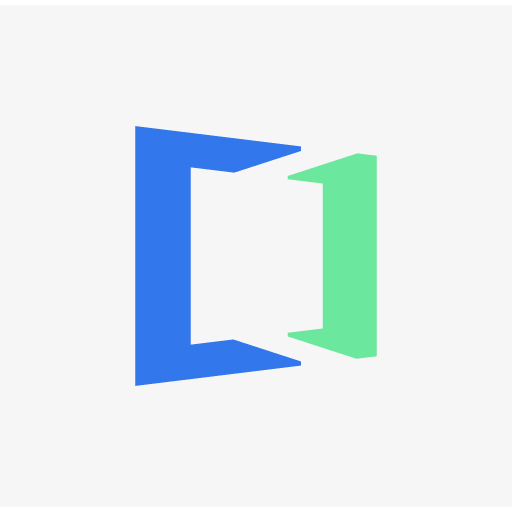
本仓将收集和展示仓颉鸿蒙应用示例代码,欢迎大家投稿,在仓颉鸿蒙社区展现你的妙趣设计!
Cangjie
247
60
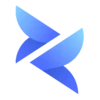
基于全新 DevUI Design 设计体系的 Vue3 组件库,面向研发工具的开源前端解决方案。
TypeScript
443
48