Node.js 中的流式文件上传库:formidable 教程
2024-12-15 19:54:14作者:尤辰城Agatha
Node.js 是一个基于 Chrome V8 引擎的 JavaScript 运行环境,广泛应用于服务器端开发。而 formidable
是一个为 Node.js 设计的用于解析 HTTP POST 请求中的文件上传的模块,它能够以流的方式处理上传的文件,高效且内存消耗小。
项目介绍
formidable
库主要解决的问题是:如何高效地处理大量文件上传以及相关数据。它具有以下特点:
- 支持大文件上传,无需将整个文件加载到内存中;
- 能够解析
multipart/form-data
的 POST 请求体; - 支持进度事件,方便跟踪上传进度;
- 提供了丰富的 API 接口,方便开发者进行定制和扩展。
项目下载位置
项目可以在 GitHub 上找到,GitHub 是目前最大的开源社区,汇集了大量优秀的开源项目。以下是 formidable
项目的 GitHub 地址:
***
项目安装环境配置
安装 formidable
之前,确保你的开发环境中已经安装了 Node.js 和 npm(Node.js 的包管理器)。以下是环境配置步骤及示例图片:
-
下载 Node.js 安装包;
-
安装 Node.js,过程中会自动安装 npm;
-
安装完成后,通过命令行检查 Node.js 和 npm 版本确保安装成功。
node -v npm -v
以下为安装环境配置成功后的命令行截图示例:
;
const http = require('http');
http.createServer((req, res) => {
if (req.url == '/upload' && req.method.toLowerCase() == 'post') {
// 解析一个文件上传
const form = new formidable.IncomingForm();
form.uploadDir = './uploads'; // 设置文件存储目录
form.parse(req, (err, fields, files) => {
if (err) {
throw err;
}
console.log('Fields:', fields);
console.log('Files:', files);
res.writeHead(200, {'content-type': 'text/plain'});
res.write('文件上传成功!');
res.end();
});
return;
}
// 显示一个文件上传界面
res.writeHead(200, {'content-type': 'text/html'});
res.end(
`<form action="/upload" enctype="multipart/form-data" method="post">
<input type="file" name="file"><br>
<input type="submit" value="Upload">
</form>`
);
}).listen(8080);
console.log('Server running at ***');
这个示例创建了一个简单的 HTTP 服务器,并在 /upload
路径上处理 POST 请求,允许用户上传文件。上传成功后,服务器会保存文件并返回成功信息。
以上就是关于 formidable
的下载、安装和基本使用教程。希望对您的项目开发有所帮助。
热门项目推荐
相关项目推荐
PDFMathTranslate
PDF scientific paper translation with preserved formats - 基于 AI 完整保留排版的 PDF 文档全文双语翻译,支持 Google/DeepL/Ollama/OpenAI 等服务,提供 CLI/GUI/DockerPython00topiam-eiam
开源IDaas/IAM平台,用于管理企业内员工账号、权限、身份认证、应用访问,帮助整合部署在本地或云端的内部办公系统、业务系统及三方 SaaS 系统的所有身份,实现一个账号打通所有应用的服务。Java00每日精选项目
🔥🔥 12.18日推荐:将文件和办公文档转换为Markdown的Python工具🔥🔥 每日推荐行业内最新、增长最快的项目,快速了解行业最新热门项目动态~~017excelize
https://github.com/xuri/excelize Excelize 是 Go 语言编写的一个用来操作 Office Excel 文档类库,基于 ECMA-376 OOXML 技术标准。可以使用它来读取、写入 XLSX 文件,相比较其他的开源类库,Excelize 支持操作带有数据透视表、切片器、图表与图片的 Excel 并支持向 Excel 中插入图片与创建简单图表,目前是 Go 开源项目中唯一支持复杂样式 XLSX 文件的类库,可应用于各类报表平台、云计算和边缘计算系统。Go02Cangjie-Examples
本仓将收集和展示高质量的仓颉示例代码,欢迎大家投稿,让全世界看到您的妙趣设计,也让更多人通过您的编码理解和喜爱仓颉语言。Cangjie038毕方Talon工具
本工具是一个端到端的工具,用于项目的生成IR并自动进行缺陷检测。Python039advanced-java
Advanced-Java是一个Java进阶教程,适合用于学习Java高级特性和编程技巧。特点:内容深入、实例丰富、适合进阶学习。JavaScript0100taro
开放式跨端跨框架解决方案,支持使用 React/Vue/Nerv 等框架来开发微信/京东/百度/支付宝/字节跳动/ QQ 小程序/H5/React Native 等应用。 https://taro.zone/TypeScript010Yi-Coder
Yi Coder 编程模型,小而强大的编程助手HTML012Community
Cangjie-TPC(Third Party Components)仓颉编程语言三方库社区资源汇总05
热门内容推荐
最新内容推荐
项目优选
收起
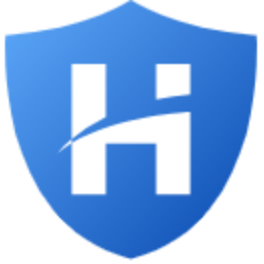
旨在打造算法先进、性能卓越、高效敏捷、安全可靠的密码套件,通过轻量级、可剪裁的软件技术架构满足各行业不同场景的多样化要求,让密码技术应用更简单,同时探索后量子等先进算法创新实践,构建密码前沿技术底座!
C
42
32
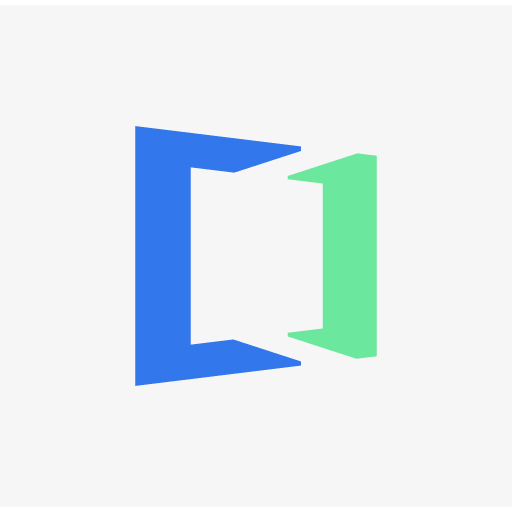
本仓将收集和展示高质量的仓颉示例代码,欢迎大家投稿,让全世界看到您的妙趣设计,也让更多人通过您的编码理解和喜爱仓颉语言。
Cangjie
166
38

🎉 基于SpringBoot,Spring Security,JWT,Vue & Element 的前后端分离权限管理系统,同时提供了 Vue3 的版本
Java
162
32
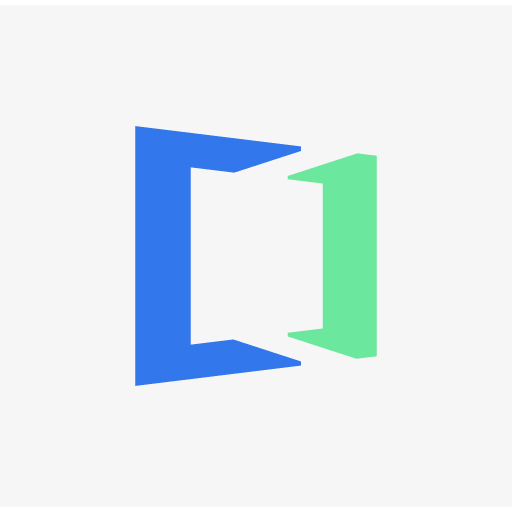
本仓将收集和展示仓颉鸿蒙应用示例代码,欢迎大家投稿,在仓颉鸿蒙社区展现你的妙趣设计!
Cangjie
248
60
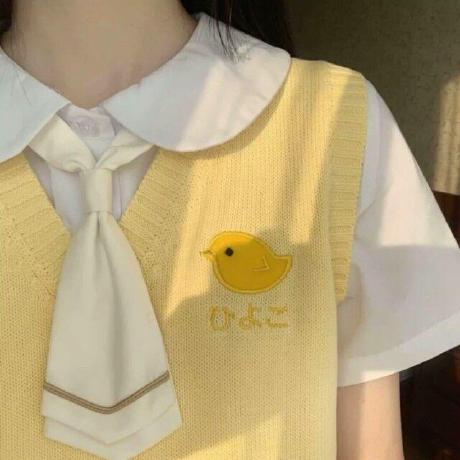
PDF scientific paper translation with preserved formats - 基于 AI 完整保留排版的 PDF 文档全文双语翻译,支持 Google/DeepL/Ollama/OpenAI 等服务,提供 CLI/GUI/Docker
Python
5
0
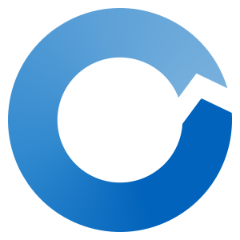
⚡️充电桩Saas云平台⚡️完整源代码,包含模拟桩模块,可通过docker编排快速部署测试。技术栈:SpringCloud、MySQL、Redis、RabbitMQ,前后端管理系统(管理后台、小程序),支持互联互通协议、市政协议、一对多方平台支持。支持高并发业务、业务动态伸缩、桩通信负载均衡(NLB)。
Java
11
9

🎉 基于Spring Boot、Spring Cloud & Alibaba、Vue3 & Vite、Element Plus的分布式前后端分离微服务架构权限管理系统
Vue
20
16
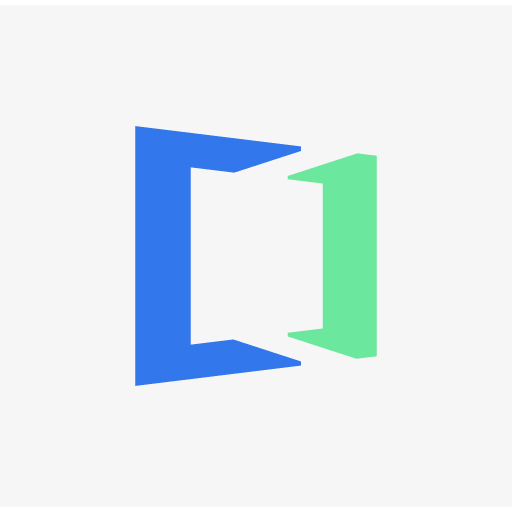
为仓颉编程语言开发者打造活跃、开放、高质量的社区环境
Markdown
892
0
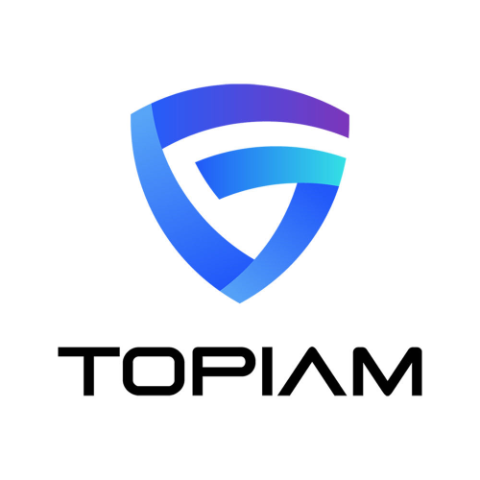
开源IDaas/IAM平台,用于管理企业内员工账号、权限、身份认证、应用访问,帮助整合部署在本地或云端的内部办公系统、业务系统及三方 SaaS 系统的所有身份,实现一个账号打通所有应用的服务。
Java
7
0
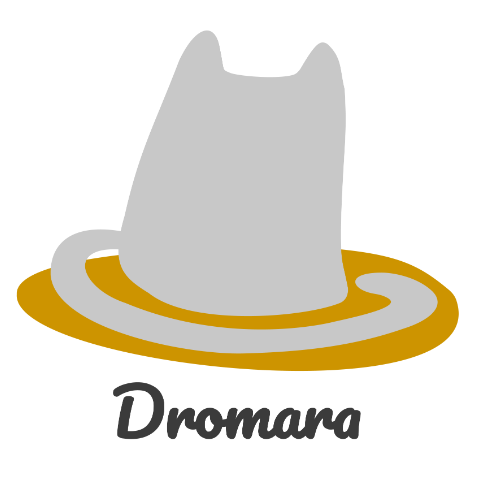
Elasticsearch
国内Top1 elasticsearch搜索引擎框架es ORM框架,索引全自动智能托管,如丝般顺滑,与Mybatis-plus一致的API,屏蔽语言差异,开发者只需要会MySQL语法即可完成对Es的相关操作,零额外学习成本.底层采用RestHighLevelClient,兼具低码,易用,易拓展等特性,支持es独有的高亮,权重,分词,Geo,嵌套,父子类型等功能...
Java
20
4