Twilio SendGrid C .NET 库技术文档
1. 安装指南
准备条件
- .NET Framework 4.0+
- .NET Core 1.0+
- .NET Standard 1.3+
- Twilio SendGrid 账户,免费注册可在前30天内发送最多40,000封邮件,之后每天可免费发送100封邮件或查看我们的定价。
获取 API Key
从 Twilio SendGrid UI 获取您的 API Key。
设置环境变量以管理 API Key
通过环境变量或 Web.config 来管理您的 Twilio SendGrid API Key,这是一个好习惯,可以将数据配置设置与代码分离。这样,您可以在不更改代码的情况下更改 Twilio SendGrid API Key。
使用 UI 设置环境变量:
- 按下 Win+R 并运行 SystemPropertiesAdvanced
- 点击环境变量
- 在用户变量部分点击新建
- 在名称中输入 SENDGRID_API_KEY(确保名称与代码中的键名称匹配)
- 在值中输入实际的 API Key
- 重启 IDE,完成设置
使用 CMD 设置环境变量:
- 以管理员身份运行 CMD
- 运行
set SENDGRID_API_KEY="YOUR_API_KEY"
以下是一些以编程方式获取和设置 API Key 的示例:
// 获取环境变量
var apiKey = Environment.GetEnvironmentVariable("SENDGRID_API_KEY");
// 设置环境变量
var setKey = Environment.SetEnvironmentVariable("SENDGRID_API_KEY", "YOUR_API_KEY");
安装包
要在您的 C# 项目中使用 Twilio SendGrid,您可以直接从我们的 GitHub 仓库 下载 Twilio SendGrid C# .NET 库,或者如果您已安装 NuGet 包管理器,可以自动获取:
dotnet add package SendGrid
# 使用 HttpClientFactory 和 Microsoft.Extensions.DependencyInjection
dotnet add package SendGrid.Extensions.DependencyInjection
安装完 Twilio SendGrid 库后,您可以在代码中调用它。有关示例实现,请参阅 .NET Core 示例 和 .NET 4.5.2 示例 文件夹。
依赖项
请查看 .csproj 文件 中的依赖项。
2. 项目使用说明
快速入门
以下是最小代码示例,用于发送简单邮件。请使用此示例,并修改 apiKey
、from
和 to
变量:
using System;
using System.Threading.Tasks;
using SendGrid;
using SendGrid.Helpers.Mail;
class Program
{
static async Task Main()
{
var apiKey = Environment.GetEnvironmentVariable("SENDGRID_API_KEY");
var client = new SendGridClient(apiKey);
var from = new EmailAddress("test@example.com", "Example User");
var subject = "Sending with Twilio SendGrid is Fun";
var to = new EmailAddress("test@example.com", "Example User");
var plainTextContent = "and easy to do anywhere, even with C#";
var htmlContent = "<strong>and easy to do anywhere, even with C#</strong>";
var msg = MailHelper.CreateSingleEmail(from, to, subject, plainTextContent, htmlContent);
var response = await client.SendEmailAsync(msg).ConfigureAwait(false);
}
}
执行上述代码后,response.StatusCode
应该为 202
,并且您应该在 to
收件人的收件箱中收到一封邮件。您可以在 UI 中查看邮件状态。或者,我们可以将事件发布到您选择的 URL,使用我们的 事件 Webhook。这将为您提供 Twilio SendGrid 处理邮件时的有关事件数据。
对于更高级的情况,您可以自己构建 SendGridMessage 对象,至少需要以下设置:
using System;
using System.Threading.Tasks;
using SendGrid;
using SendGrid.Helpers.Mail;
class Program
{
static async Task Main()
{
var apiKey = Environment.GetEnvironmentVariable("SENDGRID_API_KEY");
var client = new SendGridClient(apiKey);
var msg = new SendGridMessage()
{
From = new EmailAddress("test@example.com", "DX Team"),
Subject = "Sending with Twilio SendGrid is Fun",
PlainTextContent = "and easy to do anywhere, even with C#",
HtmlContent = "<strong>and easy to do anywhere, even with C#</strong>"
};
msg.AddTo(new EmailAddress("test@example.com", "Test User"));
var response = await client.SendEmailAsync(msg).ConfigureAwait(false);
}
}
您可以在此处找到所有邮件功能的示例。
通用 v3 Web API 使用
using System;
using System.Threading.Tasks;
using SendGrid;
class Program
{
static async Task Main()
{
var apiKey = Environment.GetEnvironmentVariable("SENDGRID_API_KEY");
var client = new SendGridClient(apiKey);
var queryParams = @"{'limit': 100}";
var response = await client.RequestAsync(method: SendGridClient.Method.GET, urlPath: "suppression/bounces", queryParams: queryParams).ConfigureAwait(false);
}
}
HttpClientFactory + Microsoft.Extensions.DependencyInjection
using System;
using System.Threading.Tasks;
using Microsoft.Extensions.DependencyInjection;
using SendGrid;
using SendGrid.Extensions.DependencyInjection;
using SendGrid.Helpers.Mail;
class Program
{
static async Task Main()
{
var services = ConfigureServices(new ServiceCollection()).BuildServiceProvider();
var client = services.GetRequiredService<ISendGridClient>();
var msg = new SendGridMessage()
{
From = new EmailAddress("test@example.com", "Example User"),
Subject = "Sending with Twilio SendGrid is Fun"
};
msg.AddContent(MimeType.Text, "and easy to do anywhere, even with C#");
msg.AddTo(new EmailAddress("test@example.com", "Example User"));
var response = await client.SendEmailAsync(msg).ConfigureAwait(false);
}
private static IServiceCollection ConfigureServices(IServiceCollection services)
{
services.AddSendGrid(options =>
{
options.ApiKey = Environment.GetEnvironmentVariable("SENDGRID_API_KEY");
});
return services;
}
}
Web 代理
var apiKey = Environment.GetEnvironmentVariable("SENDGRID_API_KEY");
var proxy = new WebProxy("http://proxy:1337");
var client = new SendGridClient(proxy, apiKey);
或者在使用 DependencyInjection 时:
services.AddSendGrid(options =>
{
options.ApiKey = Environment.GetEnvironmentVariable("SENDGRID_API_KEY");
})
.ConfigurePrimaryHttpMessageHandler(_ => new HttpClientHandler()
{
Proxy = new WebProxy(new Uri("http://proxy:1337")),
UseProxy = true
});
3. 项目 API 使用文档
请参考以下文档以获取关于 Twilio SendGrid API 的详细信息:
4. 项目安装方式
请按照以下步骤安装 Twilio SendGrid C# .NET 库:
- 通过 NuGet 包管理器自动获取:
dotnet add package SendGrid
- 使用 HttpClientFactory 和 Microsoft.Extensions.DependencyInjection:
dotnet add package SendGrid.Extensions.DependencyInjection
-
从 GitHub 仓库 下载库文件。
-
将库文件添加到您的 C# 项目中,并根据需要调用相关功能。
mybatis-plus
mybatis 增强工具包,简化 CRUD 操作。 文档 http://baomidou.com 低代码组件库 http://aizuda.comJava02open-eBackup
open-eBackup是一款开源备份软件,采用集群高扩展架构,通过应用备份通用框架、并行备份等技术,为主流数据库、虚拟化、文件系统、大数据等应用提供E2E的数据备份、恢复等能力,帮助用户实现关键数据高效保护。HTML055每日精选项目
🔥🔥 01.07日推荐:开源 LLM 友好型网络爬虫和抓取工具:Crawl4AI🔥🔥 每日推荐行业内最新、增长最快的项目,快速了解行业最新热门项目动态~~021Cangjie-Examples
本仓将收集和展示高质量的仓颉示例代码,欢迎大家投稿,让全世界看到您的妙趣设计,也让更多人通过您的编码理解和喜爱仓颉语言。Cangjie043毕方Talon工具
本工具是一个端到端的工具,用于项目的生成IR并自动进行缺陷检测。Python039PDFMathTranslate
PDF scientific paper translation with preserved formats - 基于 AI 完整保留排版的 PDF 文档全文双语翻译,支持 Google/DeepL/Ollama/OpenAI 等服务,提供 CLI/GUI/DockerPython04advanced-java
Advanced-Java是一个Java进阶教程,适合用于学习Java高级特性和编程技巧。特点:内容深入、实例丰富、适合进阶学习。JavaScript0106taro
开放式跨端跨框架解决方案,支持使用 React/Vue/Nerv 等框架来开发微信/京东/百度/支付宝/字节跳动/ QQ 小程序/H5/React Native 等应用。 https://taro.zone/TypeScript09Yi-Coder
Yi Coder 编程模型,小而强大的编程助手HTML012excelize
https://github.com/xuri/excelize Excelize 是 Go 语言编写的一个用来操作 Office Excel 文档类库,基于 ECMA-376 OOXML 技术标准。可以使用它来读取、写入 XLSX 文件,相比较其他的开源类库,Excelize 支持操作带有数据透视表、切片器、图表与图片的 Excel 并支持向 Excel 中插入图片与创建简单图表,目前是 Go 开源项目中唯一支持复杂样式 XLSX 文件的类库,可应用于各类报表平台、云计算和边缘计算系统。Go02
热门内容推荐
最新内容推荐
项目优选
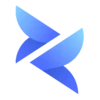
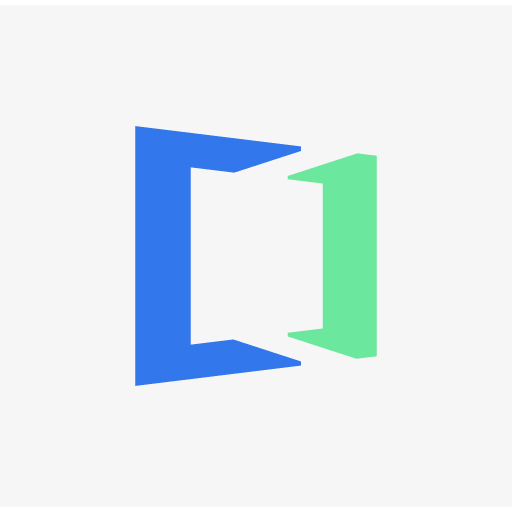
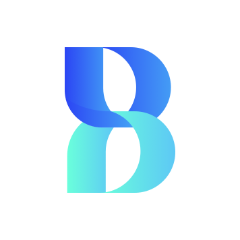
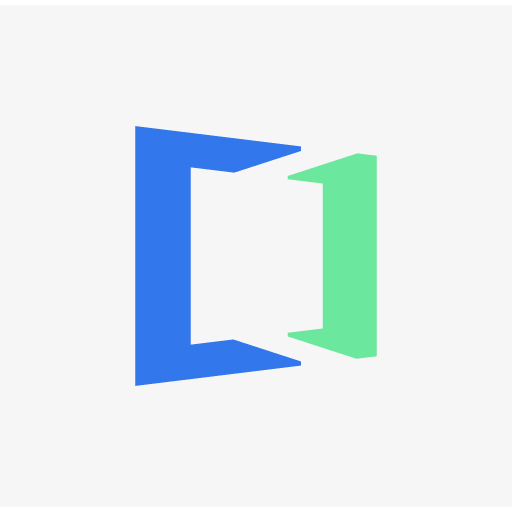
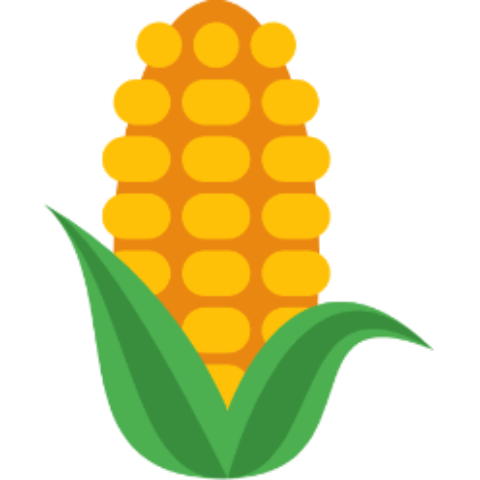

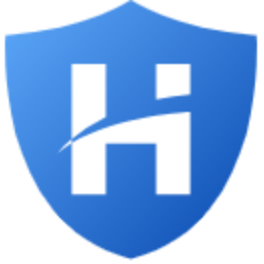
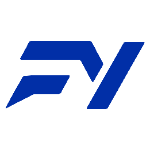

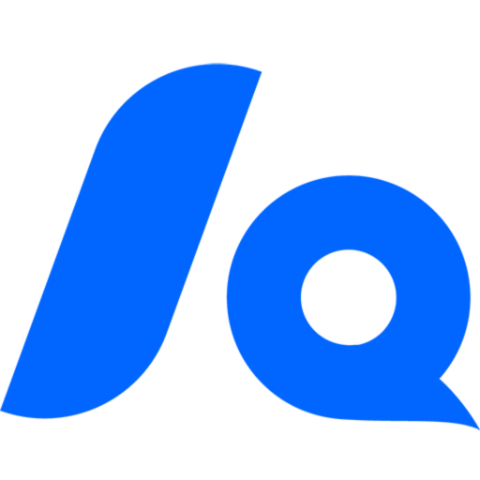