Feign Reactive 使用指南
项目介绍
Feign Reactive 是一个受 OpenFeign 启发并专为响应式编程设计的Feign客户端。该项目由 Playtika 贡献,旨在结合 Feign 的简洁API设计与 Spring WebFlux 的非阻塞、异步特性,提供高效的服务间通信解决方案。Feign Reactive 不仅让你能够利用 Feign 的优雅注解风格,还充分利用了 Spring WebClient 的优势,使你的微服务架构更加灵活且性能卓越。该库包含多个模块,如 feign-reactor-core
, feign-reactor-webclient
, 和 feign-reactor-cloud
,分别支持基础功能、WebClient集成和Spring Cloud的集成,包括负载均衡和断路器。
项目快速启动
添加依赖
首先,在你的 Spring Boot 项目中,添加以下 Maven 依赖来启用 Feign Reactive:
<dependencies>
<dependency>
<groupId>com.playtika.reactivefeign</groupId>
<artifactId>feign-reactor-cloud2</artifactId>
<version>{latest-version}</version> <!-- 替换为最新的版本号 -->
</dependency>
<dependency>
<groupId>com.playtika.reactivefeign</groupId>
<artifactId>feign-reactor-spring-configuration</artifactId>
<version>{latest-version}</version>
</dependency>
<dependency>
<groupId>com.playtika.reactivefeign</groupId>
<artifactId>feign-reactor-webclient</artifactId>
<version>{latest-version}</version>
</dependency>
</dependencies>
确保替换 {latest-version}
为实际的最新版本号。
配置Feign Client
在Spring Boot的主配置类中,启用Reactive Feign Clients:
@SpringBootApplication
@EnableReactiveFeignClients // 注意这里的"Reactive"
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
编写Feign Client接口
接下来,定义你的Feign Client接口,指定服务名和服务方法:
import reactor.core.publisher.Mono;
@FeignClient(name = "exampleService", url = "${service.example.url}")
public interface ExampleServiceClient {
@GetMapping("/hello")
Mono<String> getHello();
}
应用案例和最佳实践
异步调用实例
在服务消费端,你可以像调用本地方法那样使用定义的Feign Client,但是记得处理返回的Mono对象:
@Service
public class ConsumerService {
@Autowired
private ExampleServiceClient exampleServiceClient;
public void consumeExample() {
exampleServiceClient.getHello()
.subscribe(response -> System.out.println("Response received: " + response));
}
}
最佳实践:
- 链式调用: 利用Mono和Flux的丰富操作符进行链式调用,简化异步逻辑。
- 错误处理: 实现全局错误处理器或者在每个Feign调用处处理异常,确保良好的用户体验和系统稳定性。
- 负载均衡和断路器: 结合Spring Cloud的Ribbon和Hystrix(或CircuitBreaker),增加服务调用的健壮性。
典型生态项目
Feign Reactive天然适配Spring Cloud生态,尤其在Spring Cloud Gateway、Spring Cloud Netflix (如需向云原生过渡) 或者Spring Cloud Circuit Breaker等项目中,可以作为构建服务间通信的重要组件。通过与Spring Cloud的集成,Feign Reactive不仅能够进行服务发现,还能实现智能路由、负载均衡及断路保护等功能,非常适合构建高可用、高性能的分布式系统。
本指南简要介绍了如何开始使用Feign Reactive,进行服务间的响应式调用。实际应用中,还需根据具体需求深入探索各模块的高级特性和最佳实践,确保系统设计既高效又灵活。
CangjieCommunity
为仓颉编程语言开发者打造活跃、开放、高质量的社区环境Markdown00redis-sdk
仓颉语言实现的Redis客户端SDK。已适配仓颉0.53.4 Beta版本。接口设计兼容jedis接口语义,支持RESP2和RESP3协议,支持发布订阅模式,支持哨兵模式和集群模式。Cangjie032每日精选项目
🔥🔥 推荐每日行业内最新、增长最快的项目,快速了解行业最新热门项目动态~ 🔥🔥02qwerty-learner
为键盘工作者设计的单词记忆与英语肌肉记忆锻炼软件 / Words learning and English muscle memory training software designed for keyboard workersTSX022Yi-Coder
Yi Coder 编程模型,小而强大的编程助手HTML07advanced-java
Advanced-Java是一个Java进阶教程,适合用于学习Java高级特性和编程技巧。特点:内容深入、实例丰富、适合进阶学习。JavaScript085taro
开放式跨端跨框架解决方案,支持使用 React/Vue/Nerv 等框架来开发微信/京东/百度/支付宝/字节跳动/ QQ 小程序/H5/React Native 等应用。 https://taro.zone/TypeScript09Community
Cangjie-TPC(Third Party Components)仓颉编程语言三方库社区资源汇总05- Bbrew🍺 The missing package manager for macOS (or Linux)Ruby01
byzer-lang
Byzer(以前的 MLSQL):一种用于数据管道、分析和人工智能的低代码开源编程语言。Scala04
热门内容推荐
最新内容推荐
项目优选
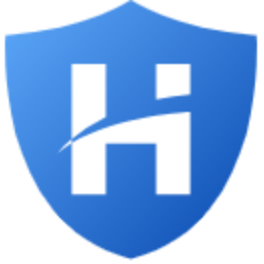
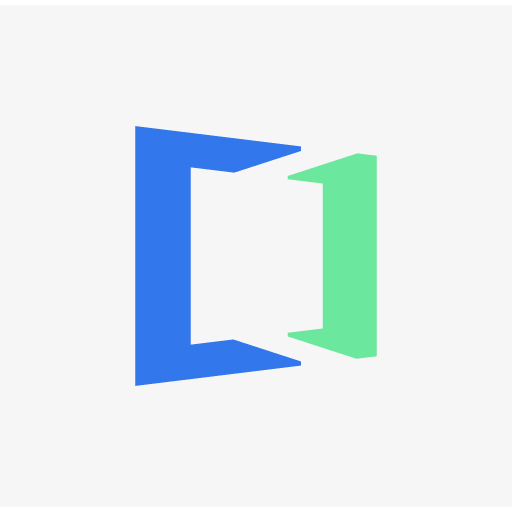
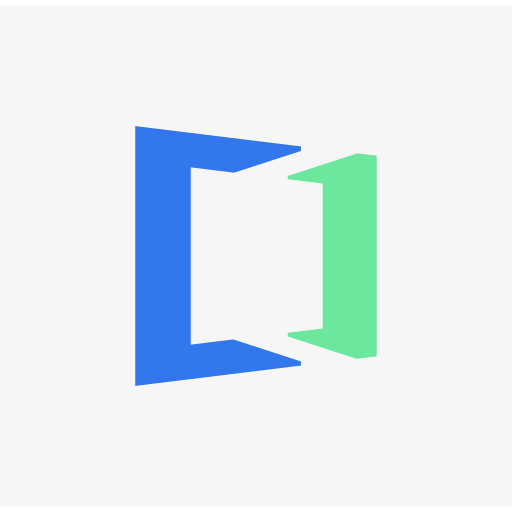
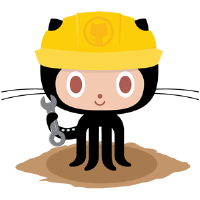
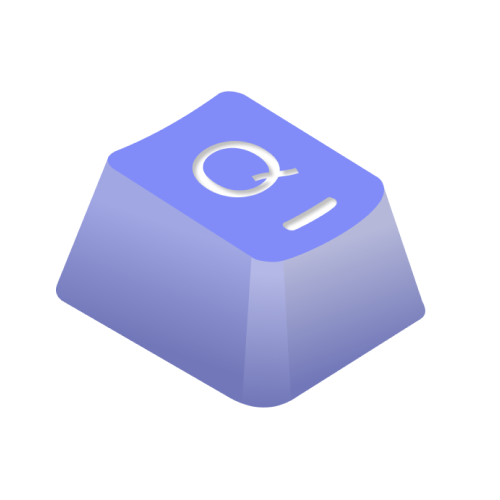
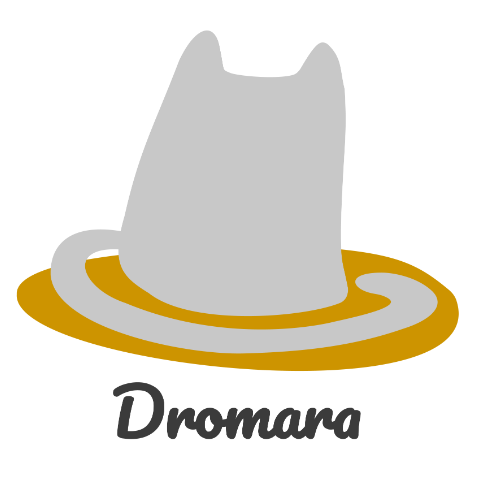
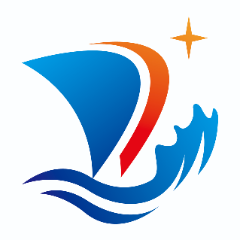
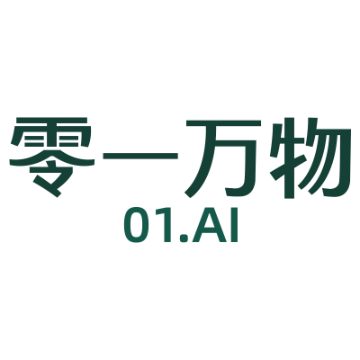

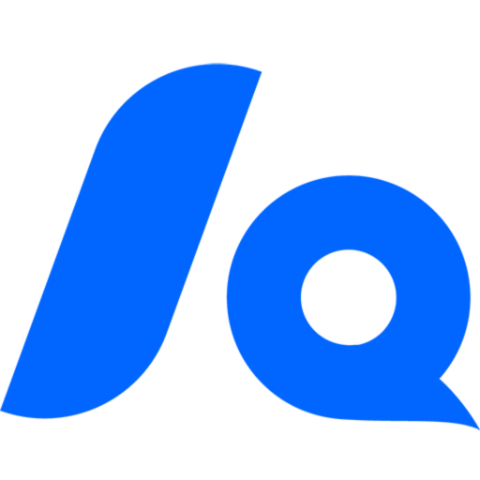