Rubyzip 项目技术文档
2024-12-17 04:48:31作者:史锋燃Gardner
1. 安装指南
1.1 系统要求
- Rubyzip 3.x 需要至少 Ruby 3.0 版本。
- Rubyzip 2.x 需要至少 Ruby 2.4 版本,并且已知在 Ruby 3.1 上可以正常工作。
- 不推荐使用早于 2.3 版本的 Rubyzip,因为存在安全问题。
1.2 安装方式
Rubyzip 可以通过 RubyGems 进行安装:
gem install rubyzip
如果你使用的是 Bundler,可以在 Gemfile
中添加:
gem 'rubyzip'
然后运行 bundle install
进行安装。
2. 项目使用说明
2.1 基本 zip 文件创建
以下是一个简单的示例,展示如何使用 Rubyzip 创建一个 zip 文件:
require 'rubygems'
require 'zip'
folder = "Users/me/Desktop/stuff_to_zip"
input_filenames = ['image.jpg', 'description.txt', 'stats.csv']
zipfile_name = "/Users/me/Desktop/archive.zip"
Zip::File.open(zipfile_name, create: true) do |zipfile|
input_filenames.each do |filename|
zipfile.add(filename, File.join(folder, filename))
end
zipfile.get_output_stream("myFile") { |f| f.write "myFile contains just this" }
end
2.2 递归压缩目录
以下示例展示了如何递归地将一个目录中的所有文件压缩到一个 zip 文件中:
require 'zip'
class ZipFileGenerator
def initialize(input_dir, output_file)
@input_dir = input_dir
@output_file = output_file
end
def write
entries = Dir.entries(@input_dir) - %w[. ..]
::Zip::File.open(@output_file, create: true) do |zipfile|
write_entries entries, '', zipfile
end
end
private
def write_entries(entries, path, zipfile)
entries.each do |e|
zipfile_path = path == '' ? e : File.join(path, e)
disk_file_path = File.join(@input_dir, zipfile_path)
if File.directory? disk_file_path
recursively_deflate_directory(disk_file_path, zipfile, zipfile_path)
else
put_into_archive(disk_file_path, zipfile, zipfile_path)
end
end
end
def recursively_deflate_directory(disk_file_path, zipfile, zipfile_path)
zipfile.mkdir zipfile_path
subdir = Dir.entries(disk_file_path) - %w[. ..]
write_entries subdir, zipfile_path, zipfile
end
def put_into_archive(disk_file_path, zipfile, zipfile_path)
zipfile.add(zipfile_path, disk_file_path)
end
end
2.3 读取 Zip 文件
以下示例展示了如何读取一个 zip 文件并提取其中的内容:
MAX_SIZE = 1024**2 # 1MiB
Zip::File.open('foo.zip') do |zip_file|
zip_file.each do |entry|
puts "Extracting #{entry.name}"
raise 'File too large when extracted' if entry.size > MAX_SIZE
entry.extract
content = entry.get_input_stream.read
end
entry = zip_file.glob('*.csv').first
raise 'File too large when extracted' if entry.size > MAX_SIZE
puts entry.get_input_stream.read
end
3. 项目 API 使用文档
3.1 Zip::File
类
3.1.1 new
方法
new
方法用于创建一个新的 zip 文件对象,支持命名参数:
def initialize(path_or_io, create: false, buffer: false, restore_ownership: DEFAULT_RESTORE_OPTIONS[:restore_ownership], restore_permissions: DEFAULT_RESTORE_OPTIONS[:restore_permissions], restore_times: DEFAULT_RESTORE_OPTIONS[:restore_times], compression_level: ::Zip.default_compression)
3.1.2 open
方法
open
方法用于打开一个现有的 zip 文件,支持命名参数:
def open(file_name, create: false, restore_ownership: DEFAULT_RESTORE_OPTIONS[:restore_ownership], restore_permissions: DEFAULT_RESTORE_OPTIONS[:restore_permissions], restore_times: DEFAULT_RESTORE_OPTIONS[:restore_times], compression_level: ::Zip.default_compression)
3.1.3 extract
方法
extract
方法用于提取 zip 文件中的条目,支持指定目标目录:
def extract(entry, entry_path = nil, destination_directory: '', &block)
3.2 Zip::Entry
类
3.2.1 new
方法
new
方法用于创建一个新的 zip 条目对象:
def initialize(zipfile, name, comment = '', extra = '', compressed_size = nil, crc = nil, compression_method = nil, size = nil, time = nil)
3.3 Zip::InputStream
类
3.3.1 get_next_entry
方法
get_next_entry
方法用于获取 zip 文件中的下一个条目:
def get_next_entry
4. 项目安装方式
4.1 通过 RubyGems 安装
gem install rubyzip
4.2 通过 Bundler 安装
在 Gemfile
中添加:
gem 'rubyzip'
然后运行 bundle install
进行安装。
5. 配置选项
5.1 覆盖现有文件
默认情况下,Rubyzip 不会覆盖已存在的文件。可以通过以下配置选项来改变这一行为:
Zip.on_exists_proc = true
5.2 非 ASCII 文件名
如果需要处理非 ASCII 文件名,可以设置以下选项:
Zip.unicode_names = true
5.3 日期验证
可以隐藏无效日期格式的警告:
Zip.warn_invalid_date = false
通过以上文档,用户可以详细了解 Rubyzip 的安装、使用、API 以及配置选项,从而更好地使用该项目。
热门项目推荐
相关项目推荐
国产编程语言蓝皮书
《国产编程语言蓝皮书》-编委会工作区017nuttx
Apache NuttX is a mature, real-time embedded operating system (RTOS).C00qwerty-learner
为键盘工作者设计的单词记忆与英语肌肉记忆锻炼软件 / Words learning and English muscle memory training software designed for keyboard workersTSX027每日精选项目
🔥🔥 01.17日推荐:一个开源电子商务平台,模块化和 API 优先🔥🔥 每日推荐行业内最新、增长最快的项目,快速了解行业最新热门项目动态~~026Cangjie-Examples
本仓将收集和展示高质量的仓颉示例代码,欢迎大家投稿,让全世界看到您的妙趣设计,也让更多人通过您的编码理解和喜爱仓颉语言。Cangjie045毕方Talon工具
本工具是一个端到端的工具,用于项目的生成IR并自动进行缺陷检测。Python039PDFMathTranslate
PDF scientific paper translation with preserved formats - 基于 AI 完整保留排版的 PDF 文档全文双语翻译,支持 Google/DeepL/Ollama/OpenAI 等服务,提供 CLI/GUI/DockerPython05mybatis-plus
mybatis 增强工具包,简化 CRUD 操作。 文档 http://baomidou.com 低代码组件库 http://aizuda.comJava03advanced-java
Advanced-Java是一个Java进阶教程,适合用于学习Java高级特性和编程技巧。特点:内容深入、实例丰富、适合进阶学习。JavaScript0108taro
开放式跨端跨框架解决方案,支持使用 React/Vue/Nerv 等框架来开发微信/京东/百度/支付宝/字节跳动/ QQ 小程序/H5/React Native 等应用。 https://taro.zone/TypeScript09
热门内容推荐
最新内容推荐
项目优选
收起
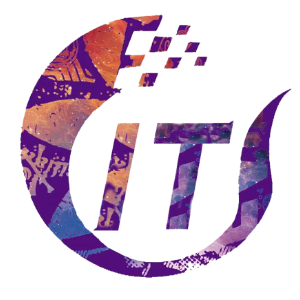
Python - 100天从新手到大师
Python
266
55
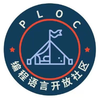
《国产编程语言蓝皮书》-编委会工作区
65
17
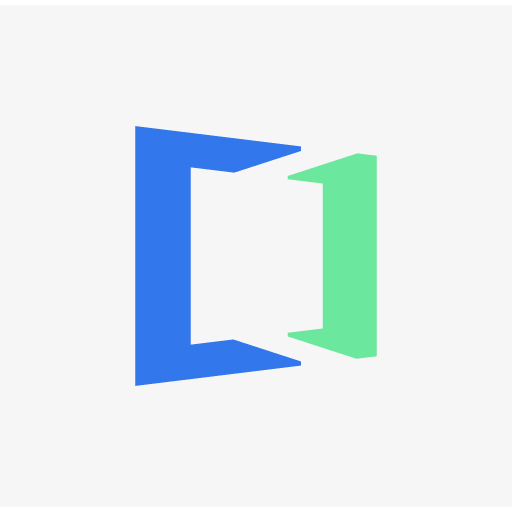
本仓将收集和展示高质量的仓颉示例代码,欢迎大家投稿,让全世界看到您的妙趣设计,也让更多人通过您的编码理解和喜爱仓颉语言。
Cangjie
196
45
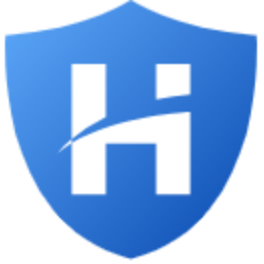
旨在打造算法先进、性能卓越、高效敏捷、安全可靠的密码套件,通过轻量级、可剪裁的软件技术架构满足各行业不同场景的多样化要求,让密码技术应用更简单,同时探索后量子等先进算法创新实践,构建密码前沿技术底座!
C
53
44
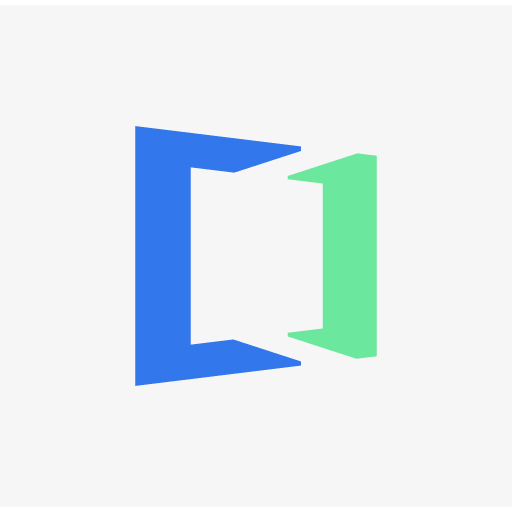
本仓将收集和展示仓颉鸿蒙应用示例代码,欢迎大家投稿,在仓颉鸿蒙社区展现你的妙趣设计!
Cangjie
268
69
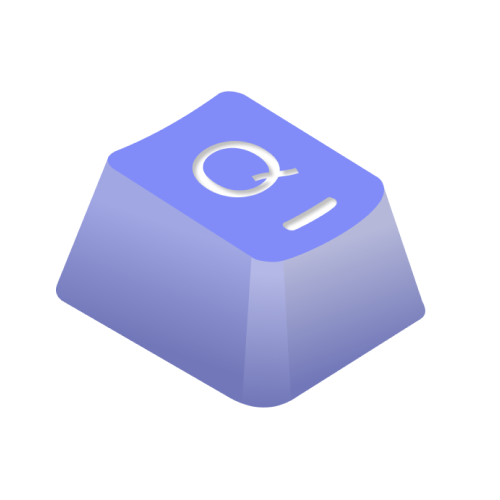
为键盘工作者设计的单词记忆与英语肌肉记忆锻炼软件 / Words learning and English muscle memory training software designed for keyboard workers
TSX
333
27
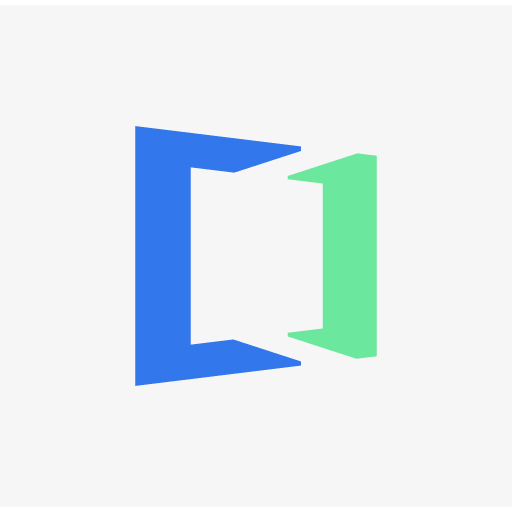
为仓颉编程语言开发者打造活跃、开放、高质量的社区环境
Markdown
896
0
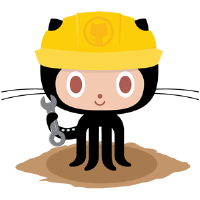
Advanced-Java是一个Java进阶教程,适合用于学习Java高级特性和编程技巧。特点:内容深入、实例丰富、适合进阶学习。
JavaScript
419
108
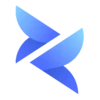
前端智能化场景解决方案UI库,轻松构建你的AI应用,我们将持续完善更新,欢迎你的使用与建议。
官网地址:https://matechat.gitcode.com
144
24
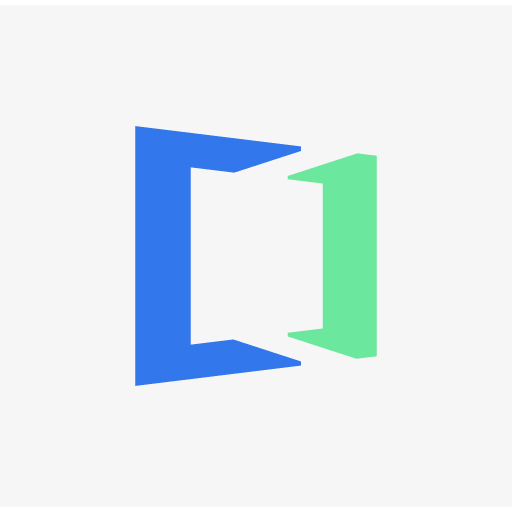
参考 HarmonyOS-Cases/Cases,提供仓颉开发鸿蒙 NEXT 应用的案例集
Cangjie
58
4